Working with SharePoint Online REST Search API 101
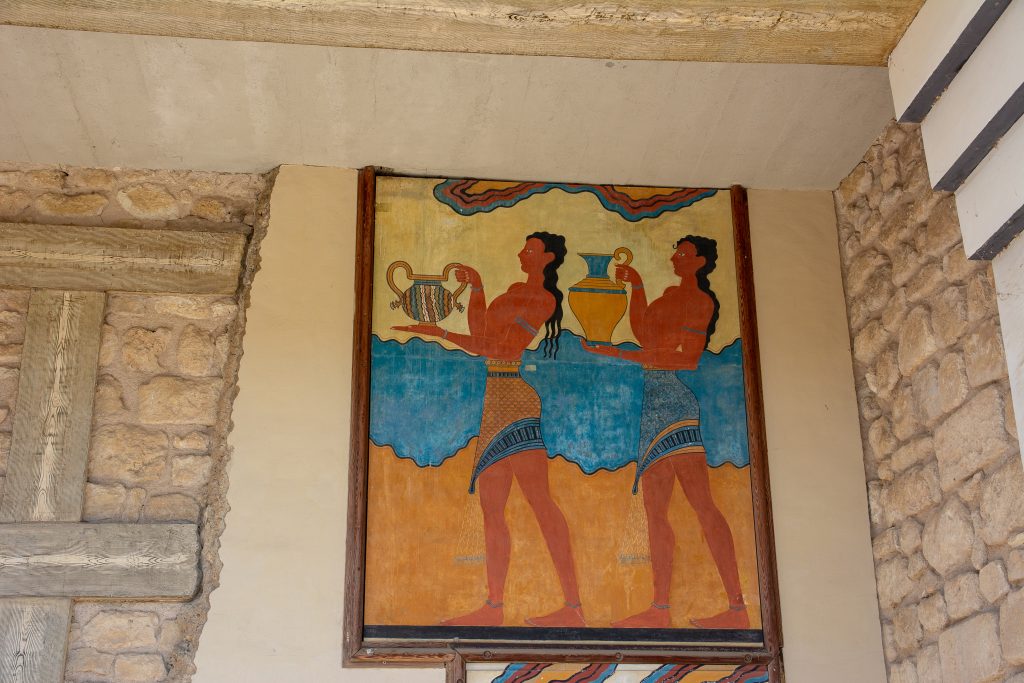
In SharePoint Online, the REST Search API is a powerful tool to query against SharePoint’s search index. It provides a comprehensive way to interact with SharePoint’s search capabilities programmatically. This blog post will guide you on how to use the SharePoint Online REST Search API to find, open, and view documents based on their metadata fields using the Graph API.
We’ll proceed in the following steps:
- Introduction to SharePoint Online REST Search API
- Authenticating with Microsoft Graph API
- Searching for Documents Based on Metadata
- Opening and Viewing Documents
You can use a tool like Postman to send these requests. The basics of sending POST or GET requests via REST API’s are not covered here, it’s expected you know how to set headers etc. as well.
Step 1: Introduction to SharePoint Online REST Search API
SharePoint Online REST Search API allows you to submit search queries and get search results programmatically. This API is useful for retrieving SharePoint data based on specific conditions or filters.
The primary endpoint of the REST Search API is /_api/search/query
. This endpoint accepts various parameters to refine the search query, and returns results in JSON format.
Step 2: Authenticating with Microsoft Graph API
To interact with the REST Search API, you must first authenticate your application with Microsoft Graph API. You can do this by registering your application in Azure Active Directory (Azure AD) and obtaining an access token.
Here’s a brief overview of the steps involved:
- Register an application in the Azure portal.
- After registration, note the
Application (client) ID
andDirectory (tenant) ID
. - Generate a new client secret under
Certificates & secrets
. - Grant API permissions for SharePoint under
API permissions
. - Get an access token. Send a POST request to the following URL:
https://login.microsoftonline.com/<tenant-id>/oauth2/v2.0/token
Replace <tenant-id>
with your Azure AD tenant ID.
The request body should include:
client_id
: your application’s Client IDscope
: “https://graph.microsoft.com/.default“client_secret
: your application’s Client Secretgrant_type
: “client_credentials”
The response will include an access_token
. Use this token to authenticate your requests to the SharePoint REST Search API. An example in PowerShell which you could easily replicate in Postman for getting a auth token:
# Define the tenant ID, client ID and secret
$tenantId = "<tenant-id>"
$clientId = "<client-id>"
$clientSecret = "<client-secret>"
# Define the resource and token endpoint
$resource = "https://graph.microsoft.com"
$tokenEndpoint = "https://login.microsoftonline.com/$tenantId/oauth2/v2.0/token"
# Prepare the request body
$body = @{
client_id = $clientId
scope = "https://graph.microsoft.com/.default"
client_secret = $clientSecret
grant_type = "client_credentials"
}
# Request the token
$response = Invoke-RestMethod -Uri $tokenEndpoint -Method Post -Body $body
# Output the access token
Write-Output "Access Token: $($response.access_token)"
Step 3: Searching for Documents Based on Metadata
You can now send authenticated GET requests to the REST Search API endpoint. The search query string goes in the querytext
parameter, and you can use other parameters to refine the search.
To find documents authored by a specific user, send a GET request to:
https://<site-url>/_api/search/query?querytext='Author:<author-name>+AND+IsDocument:True'
Replace <site-url>
with your SharePoint site URL and <author-name>
with the actual author’s name.
Include the access_token
in the Authorization
header:
GET /_api/search/query?querytext='Author:<author-name>+AND+IsDocument:True' HTTP/1.1
Host: <site-url>
Authorization: Bearer <access_token>
Accept: application/json;odata=nometadata
A script that could use the token generated above would look something like:
# Define the SharePoint Online REST Search API endpoint and query
$siteUrl = "<site-url>"
$searchEndpoint = "https://$siteUrl/_api/search/query?querytext='IsDocument:True+AND+Author:John'"
# Prepare the request headers with the access token
$headers = @{
Authorization = "Bearer $accessToken"
Accept = "application/json;odata=nometadata"
}
# Send the authenticated GET request to the SharePoint Online REST Search API
$searchResponse = Invoke-RestMethod -Uri $searchEndpoint -Method Get -Headers $headers
# Output the search results
Write-Output $searchResponse
Step 4: Opening and Viewing Documents
The search results contain various properties for each document, including the document’s URL in the Path field. To open a document, copy the Path value and paste it into a web browser.
You’ve now learned how to use the SharePoint Online REST Search API to find, open, and view documents based on their metadata fields using the Graph API. By mastering these steps, you can efficiently interact with SharePoint Online programmatically. Happy coding!