How to Create a Custom Wall Posts Webpart in SharePoint 2016
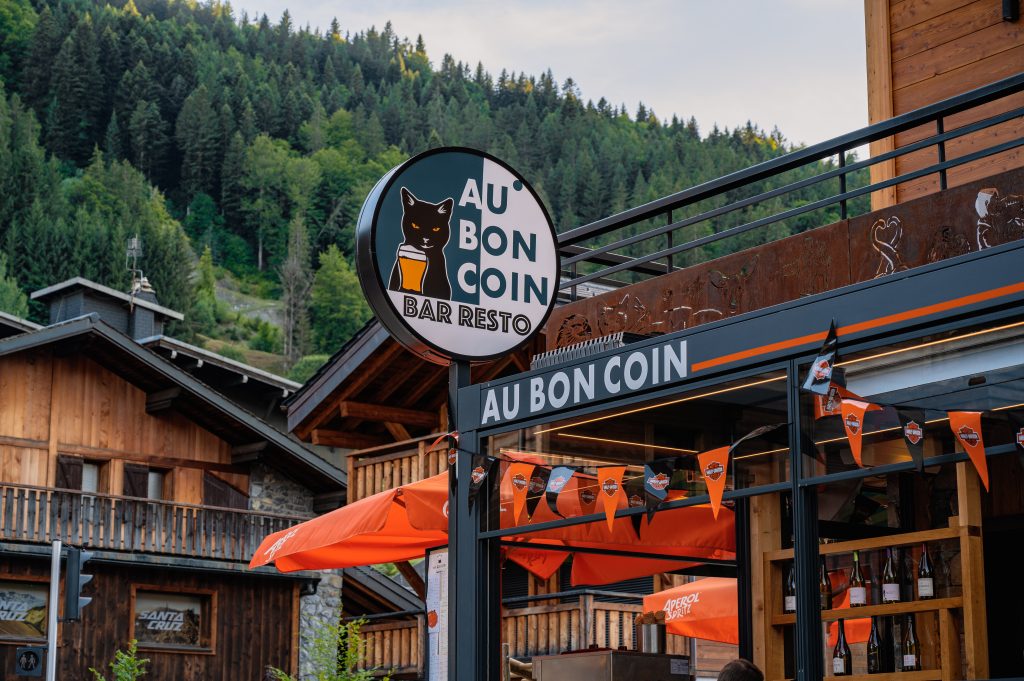
SharePoint is a versatile platform for building solutions that can fulfill various business needs. One such feature is the ability to create a custom webpart, a reusable component that can be included in SharePoint sites to provide specific functionality. Today, we’ll be discussing how to create a custom Wall Posts webpart for SharePoint 2016.
Before we begin, we should note that our custom wall posts webpart will be created using Visual Studio 2019, which supports development for SharePoint 2016. We will be writing in C# and using JavaScript and CSS for the front-end. Please ensure you have the necessary permissions to create and deploy custom webparts in your SharePoint environment.
Step 1: Create a New Project
Open Visual Studio 2019 and create a new project.
- Select File … New … Project.
- In the New Project dialog, choose Visual C# … Office/SharePoint … SharePoint Add-in. Click Next.
- Enter a name and location for your project and click Create.
- In the “What SharePoint site do you want to use for debugging?” box, enter the URL of the SharePoint site you’re developing against, and then choose “Deploy as a farm solution”. Click Finish.
Step 2: Add a New Web Part Item
Next, we’ll add a new web part to the project:
- In Solution Explorer, right-click on the project, go to Add, then New Item.
- In the Add New Item dialog, choose Visual C# … Office/SharePoint … Web Part.
- Give it a name like “WallPostsWebPart” and click Add.
Step 3: Code Your Web Part
You will now hae a new file in your project named WallPostsWebPart. This is where you’ll write the backend code for your webpart.
using System;
using System.ComponentModel;
using System.Web.UI.WebControls.WebParts;
using Microsoft.SharePoint;
using Microsoft.SharePoint.WebControls;
using System.Text;
namespace WallPostsWebPart.WallPostsWebPart
{
[ToolboxItemAttribute(false)]
public class WallPostsWebPart : WebPart
{
// Visual Studio might automatically update this path when you change the Visual Web Part project item.
private const string _ascxPath = @"~/_CONTROLTEMPLATES/15/WallPostsWebPart/WallPostsWebPart/WallPostsWebPartUserControl.ascx";
protected override void CreateChildControls()
{
Control control = Page.LoadControl(_ascxPath);
Controls.Add(control);
}
// Fetching the wall posts
public string GetWallPosts()
{
SPSite site = new SPSite("http://YourSharepointSite");
SPWeb web = site.OpenWeb();
SPList list = web.Lists["Posts"]; // This should be the name of your wall posts list
SPQuery query = new SPQuery();
query.Query = "<OrderBy><FieldRef Name='Created' Ascending='FALSE'/></OrderBy>"; // This orders the posts by creation date, newest first
SPListItemCollection items = list.GetItems(query);
StringBuilder sb = new StringBuilder();
foreach(SPListItem item in items)
{
sb.Append("<p>");
sb.Append(item["Title"].ToString()); // Assuming "Title" field holds the post content
sb.Append("</p>");
}
return sb.ToString();
}
}
}
Step 4: Create the Front-End
Now, we need to create a user control to represent the front-end of our webpart. This is done in the “WallPostsWebPartUserControl.ascx” file.
And that’s it! Now, you can deploy the solution to your SharePoint site and add the webpart to a page to test it.
<%@ Control Language="C#" AutoEventWireup="true" CodeBehind="WallPostsWebPartUserControl.ascx.cs" Inherits="WallPostsWebPart.WallPostsWebPart.WallPostsWebPartUserControl" %>
<div id="wallPostsContainer">
<%: this.GetWallPosts() %> <!-- This calls the GetWallPosts method we wrote in the code-behind -->
</div>
Step 5: Deploy the Solution
To deploy your custom webpart, follow these steps:
- In Visual Studio, on the menu, select Build … Deploy Solution.
- After the solution has been deployed, go to your SharePoint site and open a page where you want to add the webpart.
- Click on the Page tab and then Edit.
- Click on the Insert tab and then Web Part.
- In the Categories list, click Custom, find your “WallPostsWebPart”, and then click Add.
Your custom Wall Posts webpart should now be visible on the SharePoint page.
In conclusion, creating a custom webpart in SharePoint allows you to create custom functionality and provide solutions specific to your business needs. The example above showcases a simple implementation of pulling wall posts, but the possibilities are endless. You can always expand and improve the functionality based on your requirements. Happy SharePointing!