Creating a Custom SharePoint Online Webpart to Display Contact Details with Photos
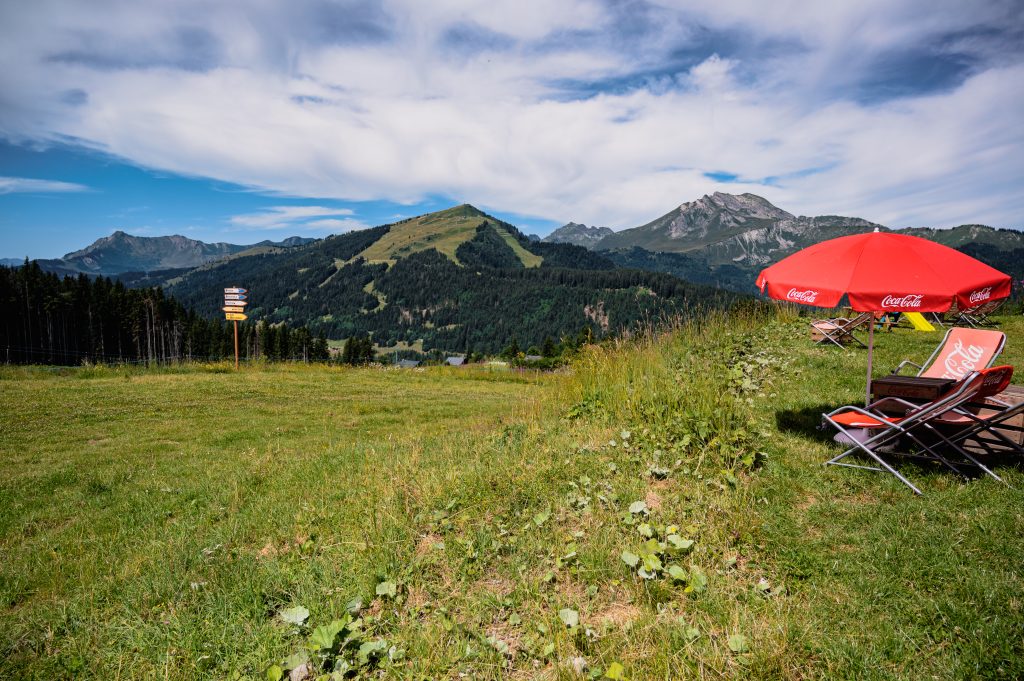
One of the powerful capabilities of SharePoint Online is its ability to create and utilize custom web parts. These web parts can help to extend the platform’s functionalities to meet specific organizational needs. In this tutorial, we will walk you through the process of creating a custom web part that displays user contact details along with their photos.
Our web part will be created using the SharePoint Framework (SPFx), which is a page and web part model for SharePoint Online. We’ll use TypeScript for the back-end, and ReactJS for the front-end. The entire process involves the following steps:
- Setting up the Development Environment
- Creating a New SharePoint Framework Solution
- Developing the Web Part
- Deploying and Testing the Web Part
Please make sure you have administrative access to the SharePoint Online and Office 365 environment. Let’s get started.
Step 1: Setting up the Development Environment
To set up your SharePoint Framework development environment, you will need Node.js, Yeoman, and the SharePoint client-side solution generator, and Gulp. Open a command prompt and run the following commands to install these dependencies:
npm install -g yo gulp
npm install -g @microsoft/generator-sharepoint
Step 2: Creating a New SharePoint Framework Solution
Now, let’s create a new SharePoint Framework solution.
Navigate to the folder where you want to create the solution, and run the following command:
yo @microsoft/sharepoint
The Yeoman SharePoint generator will start prompting you for the following information:
- What is your solution name?
- Where do you want to place the files?
- Do you want to allow the tenant admin the choice of being able to deploy the solution to all sites immediately without running any feature deployment or adding apps in sites?
- Will the components in the solution require permissions to access web APIs that are unique and not shared with other components in the tenant?
- Which type of client-side component to create?
For this example, choose the following:
- Solution Name: UserContactWebPart
- Baseline packages: Use the current folder
- Deployment option: Select Y (Yes)
- Permissions: N (No)
- Component Type: WebPart
- Web part name: UserContact
- Description: A web part that displays user contact details
- Framework: React
Step 3: Developing the Web Part
Navigate into the solution folder, and open the file src/webparts/userContact/components/UserContact.tsx
This file represents the frontend of your webpart.
You need to install the Microsoft Graph client library. To do this, run the following command:
npm install @microsoft/microsoft-graph-client
Now, let’s edit our UserContact.tsx file:
--
import * as React from 'react';
import styles from './UserContact.module.scss';
import { IUserContactProps } from './IUserContactProps';
import { escape } from '@microsoft/sp-lodash-subset';
import { MSGraphClient } from '@microsoft/sp-http';
export interface IUserContactState {
users: any[];
}
export default class UserContact extends React.Component<IUserContactProps, IUserContactState> {
constructor(props: IUserContactProps) {
super(props);
this.state = {
users: []
};
}
public componentDidMount() {
this.props.context.msGraphClientFactory
.getClient()
.then((client: MSGraphClient): void => {
// get users and their photos from Microsoft Graph
client
.api('/users')
.version('v1.0')
.get((err, res) => {
if (err) {
console.error(err);
return;
}
this.setState({ users: res.value });
});
});
}
public render(): React.ReactElement<IUserContactProps> {
return (
<div className={styles.userContact}>
{this.state.users.map((user, key) => {
return (
<div key={key}>
<h2>{user.displayName}</h2>
<p>{user.mail}</p>
<img src={`/_layouts/15/userphoto.aspx?size=L&username=${user.mail}`} alt="User photo" />
</div>
);
})}
</div>
);
}
}
Step 4: Deploying and Testing the Web Part
Now that our web part is developed, let’s deploy and test it.
To build and bundle your web part, run the following command in your command prompt:
gulp bundle --ship
And to package the solution, use the following command:
gulp package-solution --ship
These commands create a .sppkg
package in the sharepoint/solution
folder. You can upload this package to the SharePoint App Catalog in your SharePoint Online site. After uploading, you can add your web part to a page by editing the page and clicking the ‘+’ button to add a new web part. Note you’ll need to have specific permissions assigned to query Graph API.
And there you have it, a custom SharePoint Online web part that displays user contact details along with their photos. You can customize this web part further as per your needs. Happy coding!