Building SharePoint Online Remote Event Receivers for Document Review Flows
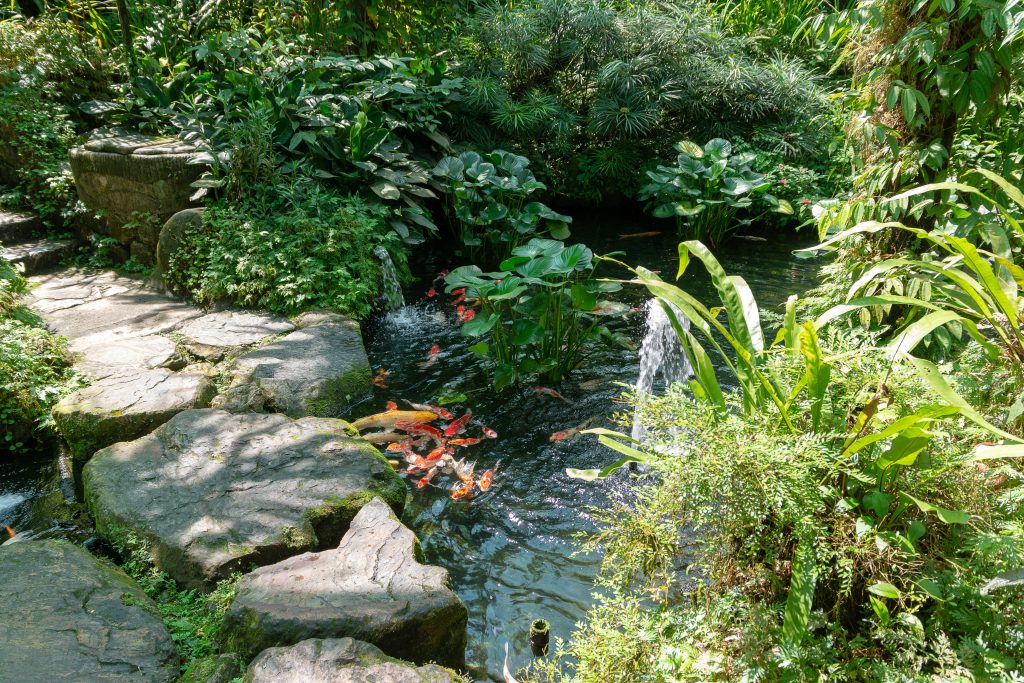
SharePoint Online has provided a myriad of collaboration and productivity tools for businesses. Among these, Remote Event Receivers and Microsoft Flow (now known as Power Automate) stand out as potent tools for automation and integration.
In this blog post, we will demonstrate how to use SharePoint Online Remote Event Receivers in combination with Power Automate to trigger a review Flow whenever a document’s status is set to “Pending” in a Document Library.
Understanding the Concepts
SharePoint Online Remote Event Receivers
Remote Event Receivers are components that handle events such as additions, updates, and deletions in SharePoint Online. Unlike their counterparts in SharePoint Server, they handle events remotely, meaning they respond to events in SharePoint Online from an external web service.
Power Automate (Flow)
Power Automate is a service offered by Microsoft that allows creation of automated workflows between apps and services to synchronise files, get notifications, collect data, and more.
Getting Started
The process is fairly straightforward and consists of the following steps:
- Creating an Azure Function
- Creating a Remote Event Receiver
- Creating a Power Automate Flow
- Attaching the Event Receiver to the Document Library
Please Note: The solution described in this post assumes you have access to and basic knowledge of Azure Functions, SharePoint Online, and Power Automate.
Step 1: Creating an Azure Function
Azure Functions is a serverless solution that allows you to write less code, maintain less infrastructure, and save costs. We’ll use it to host our Remote Event Receiver.
- Log into the Azure Portal.
- Create a new Function App.
- Once the Function App is created, create a new function within it. Use the HTTP trigger template, name your function and choose the Authorisation level (Function level should suffice).
Here is a basic example of how to structure your function:
public static async Task<HttpResponseMessage> Run(HttpRequestMessage req, TraceWriter log)
{
string content = await req.Content.ReadAsStringAsync();
log.Info(content);
// Add your code to trigger the Power Automate flow here
return req.CreateResponse(HttpStatusCode.OK);
}
This function merely logs the incoming request content and returns an OK response. You will add the logic to trigger the Power Automate flow later.
Step 2: Creating a Remote Event Receiver
In SharePoint, go to the list settings for the Document Library you want to use. Under “General Settings”, click on “Advanced settings”, and allow the management of content types by choosing “Yes”.
Now, add a new column to the Document content type. Name it “Status” and make it a Choice type field with “Pending” as one of the choices.
Once done, we will add our Remote Event Receiver. SharePoint does not provide an out-of-the-box interface to add Remote Event Receivers to lists or content types, so you will have to use the Client Side Object Model (CSOM) to attach the Event Receiver to the list.
Here is an example PowerShell script using CSOM to attach the Event Receiver to your Document Library:
# Input parameters
$siteUrl = "https://your-domain.sharepoint.com/sites/your-site"
$listTitle = "Documents"
$username = "your-username@your-domain.com"
$password = "your-password"
$securePassword = ConvertTo-SecureString -String $password -AsPlainText -Force
$credentials = New-Object -TypeName System.Management.Automation.PSCredential -ArgumentList $username, $securePassword
$addInOnly = $False
# Connect to the site
Connect-PnPOnline -Url $siteUrl -Credentials $credentials -CreateDrive
# Define the event receiver properties
$eventReceiverName = "ItemUpdated"
$eventReceiverUrl = "https://your-azure-function-url"
$eventReceiverType = [Microsoft.SharePoint.Client.EventReceiverType]::ItemUpdated
$synchronization = [Microsoft.SharePoint.Client.EventReceiverSynchronization]::Asynchronous
# Add the event receiver
Add-PnPEventReceiver -List $listTitle -Name $eventReceiverName -Url $eventReceiverUrl -EventReceiverType $eventReceiverType -Synchronization $synchronization
This script adds an ItemUpdated event receiver to your Document Library. This event receiver will trigger your Azure Function whenever an item in the Document Library is updated.
Step 3: Creating a Power Automate Flow
Next, we’ll create a Power Automate flow that will be triggered by the Azure Function whenever a document status is set to “Pending”.
- Go to Power Automate.
- Create a new Flow. Use the HTTP request trigger.
- Add a new step to send an email (or any other action you want the Flow to take).
- Save your Flow. You will be provided with an HTTP POST URL.
Step 4: Finalizing the Azure Function
Finally, we will modify the Azure Function to trigger the Power Automate flow whenever a document’s status is set to “Pending”.
- In the Azure Function, add logic to parse the incoming request content to get the item data.
- Check if the item’s status is set to “Pending”.
- If it is, send a POST request to the Power Automate flow’s URL to trigger the flow.
Here is the modified Azure Function:
public static async Task<HttpResponseMessage> Run(HttpRequestMessage req, TraceWriter log)
{
string content = await req.Content.ReadAsStringAsync();
log.Info(content);
// Parse the content as XML
var doc = new XmlDocument();
doc.LoadXml(content);
var mgr = new XmlNamespaceManager(doc.NameTable);
mgr.AddNamespace("s", "http://schemas.microsoft.com/sharepoint/soap/");
mgr.AddNamespace("z", "#RowsetSchema");
// Get the item data
var itemDataNode = doc.SelectSingleNode("//s:Changes/s:Object/s:Properties/s:SharedFields", mgr);
var itemData = itemDataNode.ChildNodes.Cast<XmlNode>().ToDictionary(n => n.LocalName, n => n.InnerText);
// Check if the item's status is set to "Pending"
if (itemData.ContainsKey("Status") && itemData["Status"] == "Pending")
{
// Trigger the Power Automate flow
var flowUrl = "https://your-power-automate-flow-url";
await new HttpClient().PostAsync(flowUrl, new StringContent(""));
}
return req.CreateResponse(HttpStatusCode.OK);
}
That’s it! You have now successfully set up a Remote Event Receiver in SharePoint Online that triggers a Power Automate flow whenever a document’s status is set to “Pending”.
This post provided a step-by-step guide on setting up this process, however, please note that this is a simplistic example and a real-world scenario would potentially be more complex.