The idea for this web part is pretty basic, take a SharePoint out of the box Posts list such as you get from an out of the box Blog Site and point a custom web part at it to retrieve posts.
Use case could be your main Intranet news feed or on your community sites. Some additional cool bits and pieces are support for custom colours via any hex codes you care to use. Plus support for custom category filtering meaning you can put multiple copies of the web part on a page, and colour code the news based on it’s category!
This is a on-premises web part, you’ll need Visual Studio and SharePoint installed to develop this. Start with a your project open and add a Visual Web Part . This doesn’t change between 2010 and 2019:
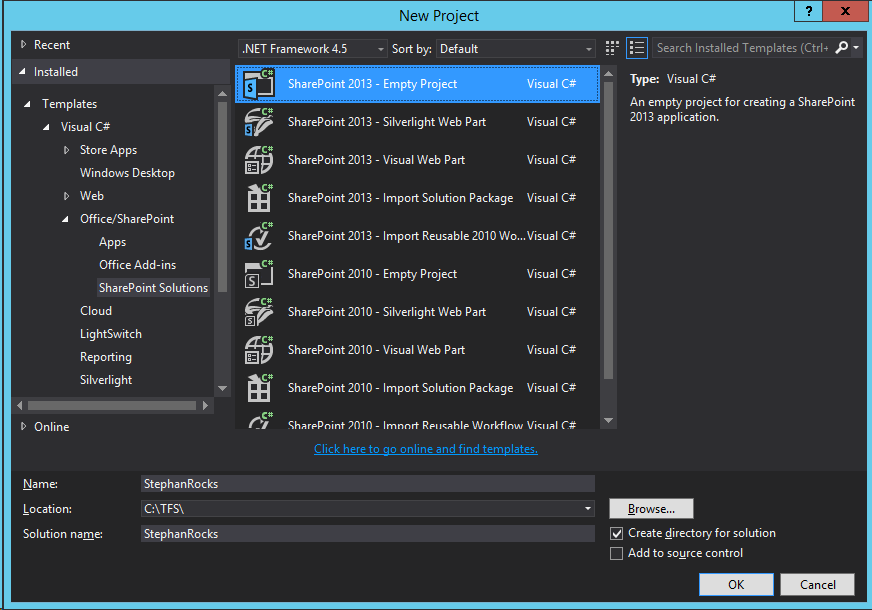
Call it whatever you like. You’ll end up with four files:
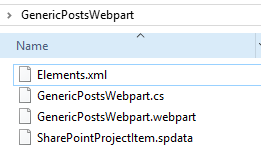
Start with the C# file. We are basically just capturing our custom properties that we want to be able to set in the web part by the end user:
using System.ComponentModel;
using System.Web.UI.WebControls.WebParts;
using YournameSpace.WebParts.CONTROLTEMPLATES.Your.Namespace;
namespace YournameSpace.WebParts.GenericPostsWebpart
{
[ToolboxItem(false)]
public class GenericPostsWebpart : WebPart
{
[WebBrowsable(true),
WebDisplayName("Enter Site URL"),
WebDescription("Enter the server relative path to the site containing your posts list"),
Personalizable(
PersonalizationScope.Shared),
Category("Custom Properties"),
DefaultValue("")]
public string siteurl
{
get;
set;
}
[WebBrowsable(true),
WebDisplayName("Enter List Name"),
WebDescription("Enter the name of the list that you want to display posts from"),
Personalizable(
PersonalizationScope.Shared),
Category("Custom Properties"),
DefaultValue("Posts")]
public string listname
{
get;
set;
}
[WebBrowsable(true),
WebDisplayName("Enter Post Limit"),
WebDescription(""),
Personalizable(
PersonalizationScope.Shared),
Category("Custom Properties"),
DefaultValue("3")]
public string postlimit
{
get;
set;
}
[WebBrowsable(true),
WebDisplayName("Enter Category (if required)"),
WebDescription("Leave blank to show all"),
Personalizable(
PersonalizationScope.Shared),
Category("Custom Properties"),
DefaultValue("")]
public string category
{
get;
set;
}
[WebBrowsable(true),
WebDisplayName("Title Link Colour"),
WebDescription("Enter the hex colour value to use for the news titles"),
Personalizable(
PersonalizationScope.Shared),
Category("Custom Properties"),
DefaultValue("#00ACB8")]
public string linkColour
{
get;
set;
}
// Visual Studio might automatically update this path when you change the Visual Web Part project item.
private const string _ascxPath = @"~/_CONTROLTEMPLATES/15/Your.Namespace/GenericPostWebpartUserControl.ascx";
protected override void CreateChildControls()
{
GenericPostWebpartUserControl control = Page.LoadControl(_ascxPath) as GenericPostWebpartUserControl;
if (control != null)
{
control.wp = this;
control.wpContainerGUID = ClientID;
control.siteurl = siteurl;
control.listname = listname;
control.postlimit = postlimit;
control.category = category;
control.linkColour = string.IsNullOrEmpty(linkColour) ? "#00ACB8" : linkColour;
Controls.Add(control);
}
}
}
}
Your elements XML file is next up, all you really need to do is set the group and title properties to what you want:
<?xml version="1.0" encoding="utf-8"?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/" >
<Module Name="GenericPostsWebpart" List="113" Url="_catalogs/wp">
<File Path="GenericPostsWebpart\GenericPostsWebpart.webpart" Url=" YournameSpace.WebParts_GenericPostsWebpart.webpart" Type="GhostableInLibrary">
<Property Name="Group" Value="Custom" />
<Property Name="Title" Value="Generic Posts" />
</File>
</Module>
</Elements>
The webpart file can be customised next, again the title and description are the parts your end users will see:
<?xml version="1.0" encoding="utf-8"?>
<webParts>
<webPart xmlns="http://schemas.microsoft.com/WebPart/v3">
<metaData>
<type name="YournameSpace.WebParts.GenericPostsWebpart.GenericPostsWebpart, $SharePoint.Project.AssemblyFullName$" />
<importErrorMessage>$Resources:core,ImportErrorMessage;</importErrorMessage>
</metaData>
<data>
<properties>
<property name="Title" type="string">GenericPostsWebPart</property>
<property name="Description" type="string">Displays blog type articles from any list</property>
</properties>
</data>
</webPart>
</webParts>
This should get you a working web part that you can deploy to your test environment. What will it look like? What per-requisites is this web part expecting? First you’ll want a test site, with a subsite setup I’ve called mine ‘News’. This subsite was made ‘Blog’ template so it has a ‘Posts’ list already but you can just create one manually if you like.
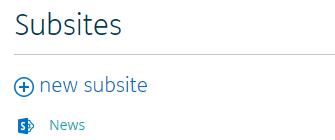
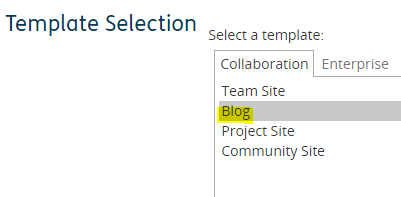
Now on the parent site (not the ‘News’ subsite) add to any page your custom web part and configure it!
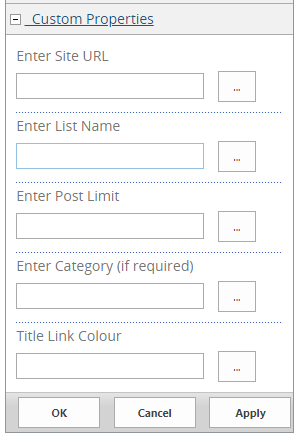
Site URL should be relative so something like /community/mysite/news
List name should be ‘Posts’ if you are using the out of the box Blog Site template otherwise point it at whatever custom list you created using that content type.
The post limit can be whatever you like 1 to 3 is usually good.
Category is actually just looking at the out of the box category column, use it to filter your news posts if you like. Works well with the colour coding.
Link colour is expecting a HEX colour code. Any HEX colour code will work.
What does this all result in? A neat little summary web part like so (text truncated):
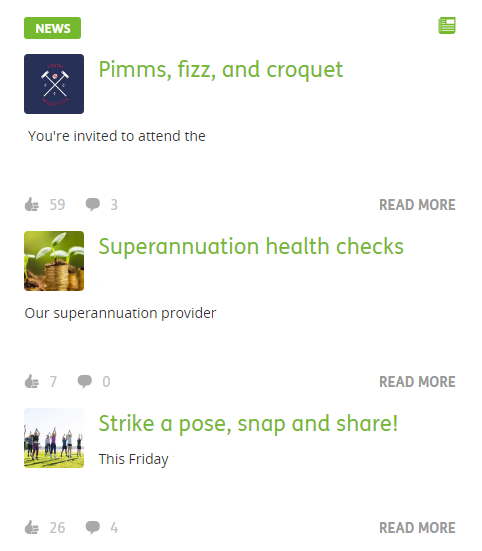

The articles are clickable and open in a modal dialog, they provide all the social likes and comments functionality you get with the ‘Posts’ list and SharePoint 2013 and newer.
The modal dialogs contain the full article, the buttons show only if you have edit rights (these are custom and will be covered in another article):
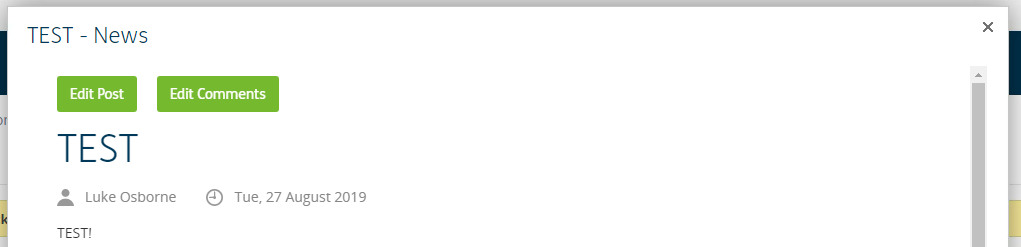
At the bottom of each modal dialog your users can like and comment on these posts.

So there you have it, a simple re-usable generic posts web part. Use it however you will. The screenshots were done using a custom master page, the modal dialogs that open are also not the out of the box ones they’ve been customised too. I’ll hopefully write up all of that and how it works in the future.
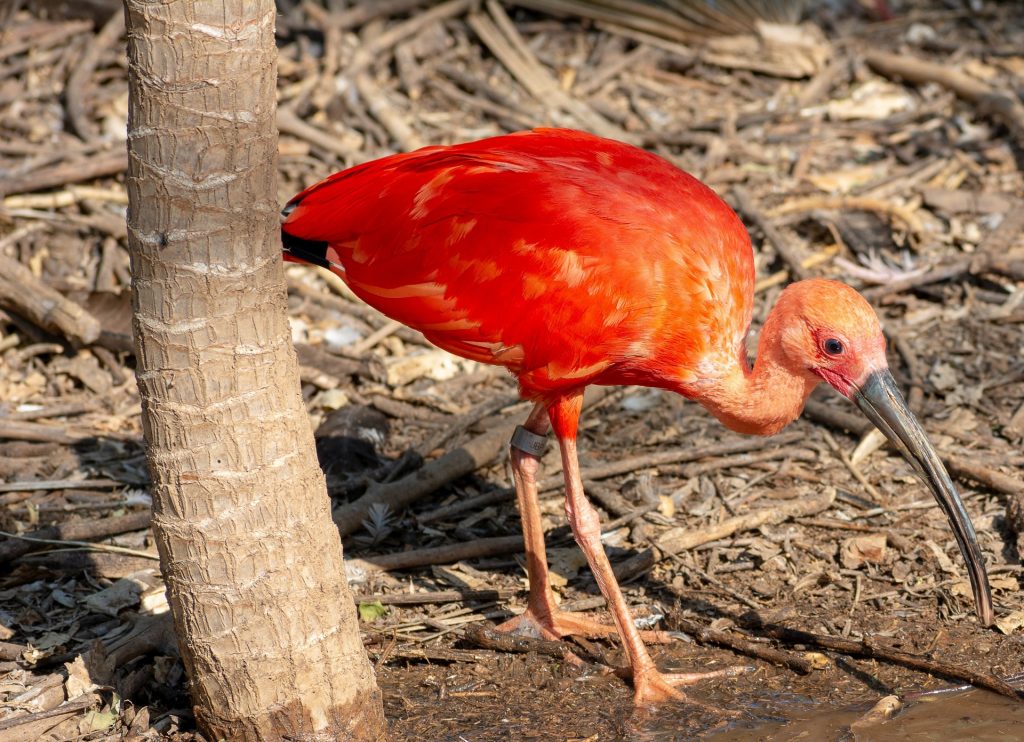
Thanks for reading my blog posts, they are really just a way to capture what I do in day to day life.